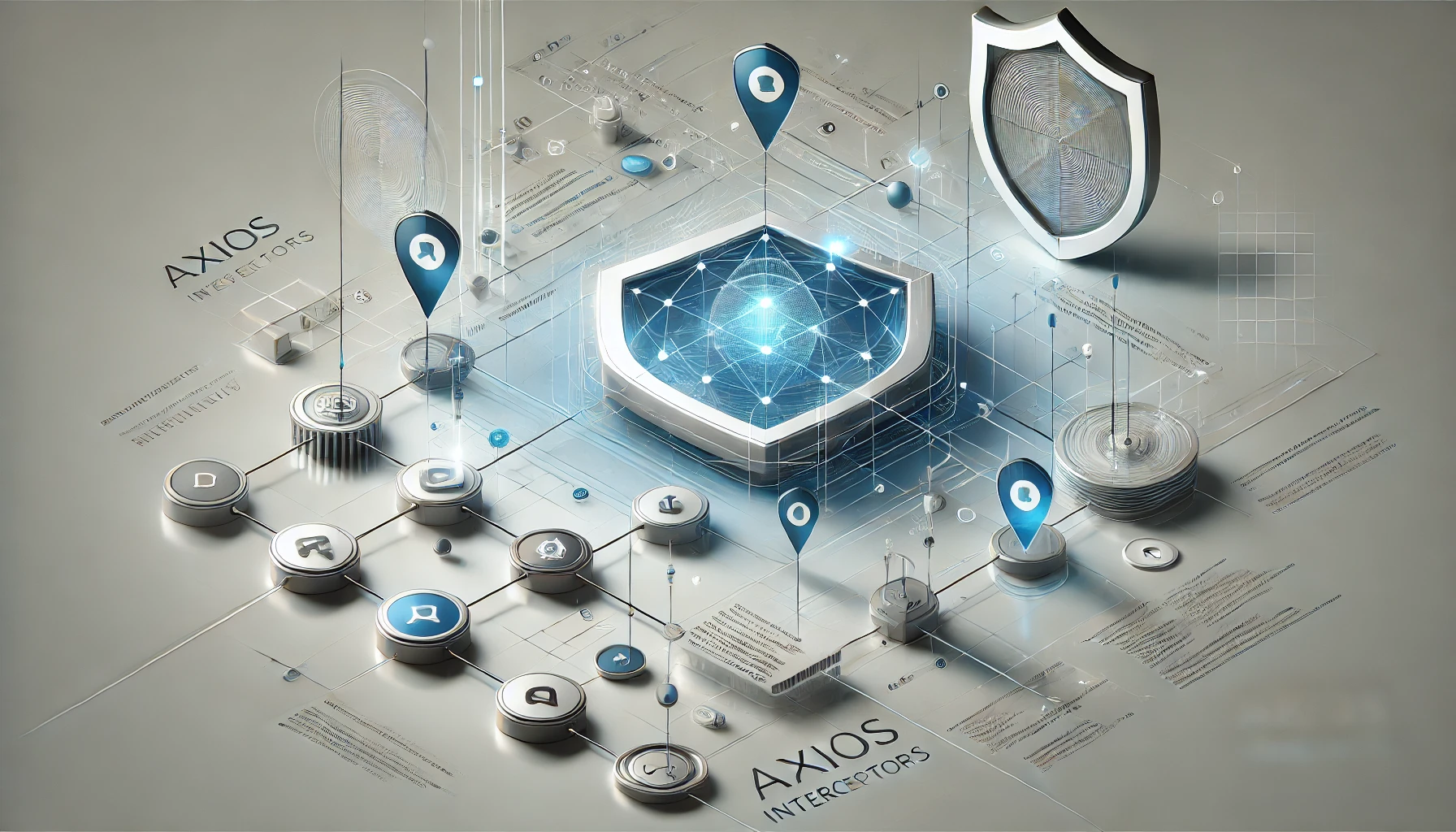
Axios Interceptors
Shamal Iroshan
2023-05-05 | 9 min read
Axios is a popular JavaScript library used for making HTTP requests from web browsers or Node.js. It provides an easy-to-use and efficient way to interact with APIs and fetch data from servers. With Axios, you can perform various types of requests, such as GET, POST, PUT, and DELETE, and handle the server responses in a convenient manner. It supports features like request and response interception, automatic transformation of JSON data, setting request headers, handling errors, and more. Axios is widely used in web development for its simplicity, versatility, and compatibility with modern JavaScript frameworks and environments.
What is Axios
Axios is a versatile and widely used JavaScript library that simplifies the process of making HTTP requests from web browsers or Node.js applications. It provides an intuitive and efficient way to interact with APIs and retrieve data from servers. With Axios, developers can perform various types of requests, including GET, POST, PUT, and DELETE, by leveraging its clean and straightforward API.
One of the key advantages of using Axios is its ease of use. The library offers a simple and consistent interface that allows developers to make HTTP requests with minimal code. It abstracts away the complexities of handling XMLHttpRequest or Fetch API directly, providing a more user-friendly experience. Additionally, Axios supports promises, making it easy to handle asynchronous operations and work with data asynchronously.
Axios also provides a range of convenient features that enhance the request and response handling process. For instance, it supports request and response interception, allowing developers to modify or inspect requests and responses before they are sent or processed. This enables the implementation of custom logic, such as adding authentication headers or handling caching at the request level.
Furthermore, Axios seamlessly handles JSON data by automatically converting request and response data to JSON format. It simplifies the process of sending and receiving JSON payloads, eliminating the need for manual conversion. Additionally, Axios provides extensive error-handling capabilities, enabling developers to catch and handle errors in a graceful manner. This includes handling common HTTP error status codes and network-related errors.
Due to its simplicity, versatility, and compatibility with modern JavaScript frameworks and environments, Axios has become a go-to choice for many developers in web development projects. It empowers developers to easily communicate with APIs, retrieve data from servers, and handle responses efficiently. Its popularity is attributed to its straightforward API, comprehensive feature set, and the overall developer-friendly experience it offers when working with HTTP requests.
you can simply add Axios to your project,
npm install axios
or
yarn add axios
What are Interceptors
Axios interceptors are a powerful feature that allows you to intercept and manipulate HTTP requests and responses made by Axios. They provide a convenient way to add global logic or modify data before the requests are sent or after the responses are received.
Axios interceptors consist of two types: request interceptors and response interceptors. Request interceptors are functions that are executed before a request is sent, giving you the opportunity to modify the request config or headers, add authentication tokens, or perform any other necessary pre-request actions. Response interceptors, on the other hand, are functions that are executed after a response is received, allowing you to modify the response data, handle errors, or implement custom logic based on the response.
Interceptors can also be used to handle errors. By adding a response interceptor and checking the status code of the response, you can define error-handling logic based on specific conditions. Additionally, you can use interceptors to automatically refresh authentication tokens, transform response data, log requests and responses, or perform any other necessary operations.
Axios interceptors provide a convenient way to centralize and customize behavior across your application when making HTTP requests. They help keep your codebase clean and maintainable by separating concerns related to request/response manipulation and global logic. With interceptors, you have fine-grained control over the request/response lifecycle and can easily implement custom functionality without scattering it throughout your codebase.
Request Interceptors
Axios request interceptors are functions that are executed before a request is sent. They provide a way to intercept and modify the request configuration or headers, add authentication tokens, handle errors, or perform any other necessary actions before the request is dispatched.
Request interceptors in Axios are registered using the
axios.interceptors.request.use()
method. This method takes two parameters: a callback function to be executed before each request and an optional error callback function. The callback function receives the request configuration object as a parameter and can modify it or return a new configuration object. The error callback function is triggered if an error occurs during the interceptor execution.
Here's an example of how to use a request interceptor in Axios:
axios.interceptors.request.use(
function (config) {
// Modify request configuration or headers
config.headers.Authorization = 'Bearer ' + getToken();
// Perform other pre-request actions
return config; // Return modified or new configuration
},
function (error) {
// Handle request interceptor error
return Promise.reject(error);
}
);
In this example, the request interceptor modifies the headers by adding an authentication token retrieved from the
getToken()
function. You can perform any necessary modifications to the request configuration, such as adding headers, changing the URL, or manipulating the payload.
It's important to note that request interceptors can also return a Promise. This allows for asynchronous operations, such as making additional API calls to fetch data before the request is sent.
Axios request interceptors are powerful tools that enable you to add custom logic, manipulate requests, and handle errors uniformly across your application. They provide a central point to modify outgoing requests and can help streamline common request-related operations.
Response Interceptors
Axios response interceptors are functions that are executed after a response is received from the server. They allow you to intercept and modify the response data, handle errors, implement custom logic based on the response, or perform any other necessary actions.
Response interceptors in Axios are registered using the
axios.interceptors.response.use()
method. This method takes two parameters: a callback function to be executed after each successful response and an optional error callback function. The callback function receives the response object as a parameter and can modify the response data or return a new response object. The error callback function is triggered if an error occurs during the interceptor execution.
Here's an example of how to use a response interceptor in Axios:
axios.interceptors.response.use(
function (response) {
// Modify or process response data
const modifiedResponse = doSomething(response);
// Perform other post-response actions
return modifiedResponse; // Return modified or new response object
},
function (error) {
// Handle response interceptor error
return Promise.reject(error);
}
);
In this example, the response interceptor applies some modifications or additional processing to the received response data using the
doSomething()
function. You can perform any necessary manipulations or custom logic based on the response, such as transforming the data, handling errors, logging, or implementing caching mechanisms.
Similar to request interceptors, response interceptors can also return Promises, allowing for asynchronous operations, such as making additional API calls or fetching additional data based on the response.
Axios response interceptors provide a convenient way to centralize and customize behavior for handling responses across your application. They enable you to modify response data, handle errors uniformly, implement global logic based on the received responses, and maintain a clean and maintainable codebase.
Example of Managing JWT Token
Here's an example of how you can use Axios interceptors to manage JWT token regeneration
import axios from 'axios';
// Create an Axios instance
const axiosInstance = axios.create({
baseURL: 'https://api.example.com',
});
// Function to refresh the JWT token
async function refreshJWTToken() {
try {
// Make an API call to refresh the token
const response = await axiosInstance.post('/refresh-token', {
// Include any necessary data for token refresh
});
// Extract the new token from the response
const newToken = response.data.token;
// Save the new token to the token storage (e.g., local storage, state management)
saveToken(newToken);
// Return the new token
return newToken;
} catch (error) {
// Handle token refresh error
throw error;
}
}
// Request interceptor to add JWT token to outgoing requests
axiosInstance.interceptors.request.use(
function (config) {
const token = getToken();
if (token) {
// Add the JWT token to the request headers
config.headers.Authorization = `Bearer ${token}`;
}
return config;
},
function (error) {
return Promise.reject(error);
}
);
// Response interceptor to handle token expiration and regeneration
axiosInstance.interceptors.response.use(
function (response) {
// Return the response as-is
return response;
},
async function (error) {
const originalRequest = error.config;
if (error.response && error.response.status === 401 && !originalRequest._retry) {
originalRequest._retry = true;
try {
// Refresh the JWT token
const newToken = await refreshJWTToken();
// Update the original request with the new token
originalRequest.headers.Authorization = `Bearer ${newToken}`;
// Retry the original request
return axiosInstance(originalRequest);
} catch (refreshError) {
// Handle token refresh error
return Promise.reject(refreshError);
}
}
return Promise.reject(error);
}
);
In this example, we create an Axios instance called axiosInstance with a base URL. We define a refreshJWTToken function that makes an API call to refresh the JWT token. The response interceptor checks for a 401 (Unauthorized) error response and, if encountered, triggers the token regeneration process by calling the refreshJWTToken function. It then updates the original request with the new token and retries the request. If the token refresh fails or encounters an error, it propagates the error downstream.
The request interceptor adds the JWT token to the request headers before sending the request, ensuring that the authenticated user's token is included in the API calls.
By using Axios interceptors in this manner, you can seamlessly handle token expiration, regenerate tokens, and automatically update the headers for subsequent requests, providing a smooth and secure user experience.