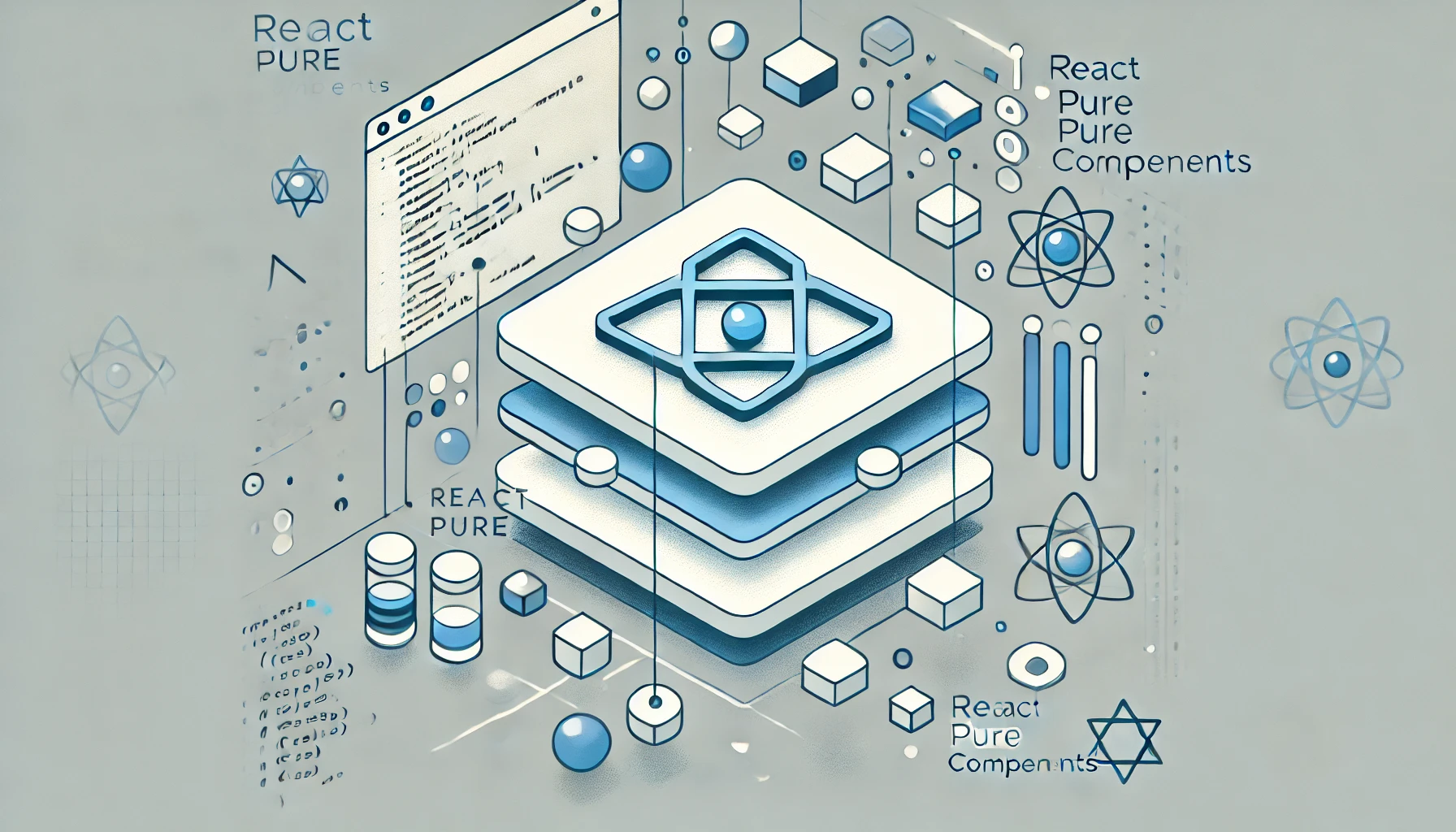
React pure components
Shamal Iroshan
2021-07-21 | 2 min read
From this post, I'm going to talk about what is a react pure component and when we can use it.
React component
The component in react is reusable code that is the same as javascript functions, but different with these two is react components return HTML elements via render method.
If we use state or props with react component when these state or props value is changed react component does not care that the changed value is same or different either way component is going to rerender. this can cause to lack of performance on the website.
To avoid this issue we can use the shouldComponentUpdate method from this method we can check whether the state or props changed or not. if it is changed we can update our component.
class CounterButton extends React.Component {
constructor(props) {
super(props);
this.state = {count: 1};
}
shouldComponentUpdate(nextProps, nextState) {
if (this.props.color !== nextProps.color) {
return true;
}
if (this.state.count !== nextState.count) {
return true;
}
return false;
}
render() {
return (
<button
color={this.props.color}
onClick={() => this.setState(state => ({count: state.count + 1}))}
>
Count: {this.state.count}
</button>
);
}
}
we can use this for a simple component, but what will happen if our component gets more complex. 🤔
React pure component
This is the place that reacts pure components will come in handy.
Pure components in react do not go to re-render if the state or props are updated with the same values. this will also ensure the high performance of react component.
Features of react pure component.
- Prevents re-rendering of Component if props or state is the same
- Takes care of “shouldComponentUpdate” implicitly
- State and Props are Shallow Compared
- Pure Components are more performant in certain cases
This pure component is the same as react Component difference is pure component takes care of the shouldComponentUpdate method.
we need to remember that state and props are only shallow compared.
class CounterButton extends React.PureComponent {
constructor(props) {
super(props);
this.state = {count: 1};
}
render() {
return (
<button
color={this.props.color}
onClick={() => this.setState(state => ({count: state.count + 1}))}
>
Count: {this.state.count}
</button>
);
}
}
Now because the above component is extended with react pure component it is only updated if state or props value changed to different values.
Hope you get something useful from this post.