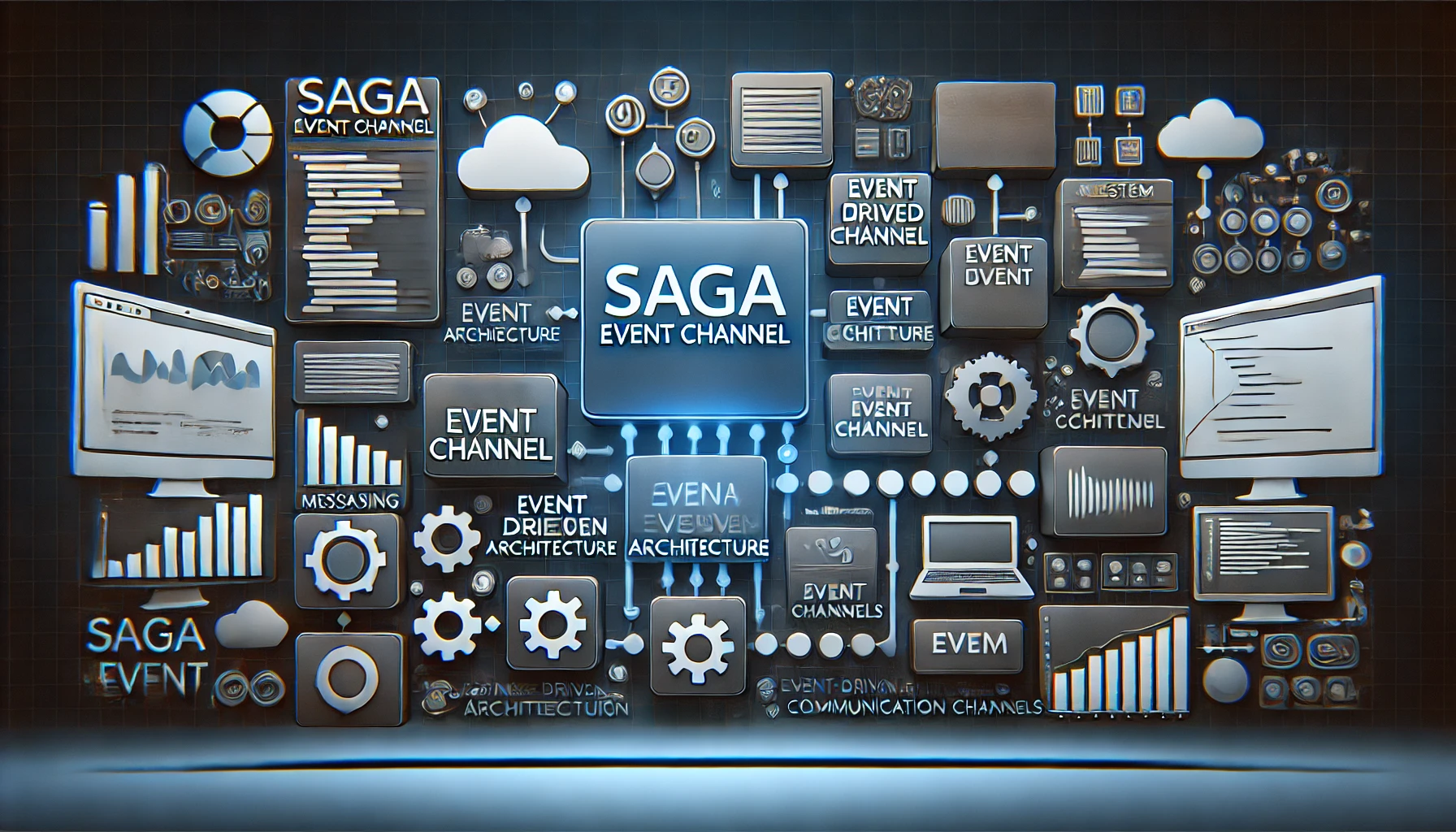
Saga Event Channel
Shamal Iroshan
2023-05-01 | 4 min read
saga is a middleware library called Redux Saga that is commonly used with Redux for managing asynchronous behavior in React applications. Redux Saga provides a way to handle complex asynchronous operations, such as making API calls, handling side effects, and managing application flow in a more organized and declarative manner. It utilizes generator functions, known as sagas, to encapsulate the logic for handling these asynchronous operations. Sagas enable developers to write readable, testable, and maintainable code by separating the side effect logic from the components and reducers. With Redux Saga, developers can orchestrate complex asynchronous workflows, handle data fetching and updating, and manage application states more effectively in React applications.
What is Saga Event Channel
Redux Saga Event Channel is a powerful feature provided by Redux Saga that allows you to create and manage long-lived communication channels for handling asynchronous events in your Redux-based application. Event channels act as event streams that can emit and receive events from various sources, such as WebSocket connections, Web Workers, or any other event source.
With Redux Saga Event Channel, you can define how events are received, transformed, and dispatched to your Redux store. It provides a declarative way to handle event-based asynchronous operations, enabling you to manage complex event flows and side effects.
Event channels are created using the eventChannel
function provided by Redux Saga. This function takes a subscriber function as an argument, which is responsible for subscribing to the event source and emitting events. The subscriber function should return a cleanup function that is called when the event channel is closed.
Once created, you can use event channels with Saga effects like take, put, and call to handle incoming events, dispatch Redux actions, and perform other side effects. You can also cancel or close event channels to stop listening to events and clean up resources.
Redux Saga Event Channel provides a powerful and flexible mechanism for managing complex event-driven asynchronous flows within your Redux application. It allows you to integrate external event sources seamlessly and handle asynchronous operations in a centralized and controlled manner, resulting in more maintainable and scalable code.
Usage of Saga Event Channel
Import the necessary functions: In the file where you want to use Event Channels, import the required functions from the Redux Saga library
import { eventChannel, take, put, call } from 'redux-saga/effects';
Define a function that creates an Event Channel using the eventChannel function. This function should handle the subscription to the event source and emit events.
function createEventChannel() {
return eventChannel((emit) => {
// Subscribe to the event source and emit events as necessary
const eventSource = new EventSource('your-event-source-url');
eventSource.onmessage = (event) => {
// Emit the event to the Event Channel
emit(event.data);
};
// Return the cleanup function to be called when the channel is closed
return () => {
eventSource.close();
};
});
}
By following these steps, you can create and use Event Channels with Redux Saga in your application. The Event Channel will listen to events from a specified event source, and the Saga will handle those events and dispatch appropriate Redux actions. This allows you to manage asynchronous event-driven flows and side effects in a centralized and controlled manner.
Here's an example of how you can use Redux Saga Event Channels to track the progress of a file upload
Define an Event Channel for Progress Updates
import { eventChannel } from 'redux-saga';
import { call, put, take } from 'redux-saga/effects';
function createProgressEventChannel(file) {
return eventChannel((emit) => {
const onProgress = (progressEvent) => {
if (progressEvent.lengthComputable) {
const progress = Math.round((progressEvent.loaded / progressEvent.total) * 100);
emit(progress);
}
};
// Start tracking progress using the appropriate file upload mechanism
const uploadTask = uploadFile(file, onProgress);
// Return the cleanup function to be called when the channel is closed
return () => {
// Clean up any ongoing file upload or event listeners
uploadTask.cancel();
};
});
}
Create a Saga to Handle Progress Updates
function* uploadProgressSaga(action) {
const { file } = action.payload;
const channel = yield call(createProgressEventChannel, file);
while (true) {
const progress = yield take(channel);
// Dispatch an action to update the file upload progress in the Redux store
yield put({ type: 'UPLOAD_PROGRESS_UPDATED', payload: progress });
}
}
In this example, the createProgressEventChannel
function creates an Event Channel that listens to progress events emitted during the file upload. The progress is calculated based on the progressEvent
object received during the upload process. The Event Channel is created using the eventChannel
function from Redux Saga.
The uploadProgressSaga
is a Saga generator function that listens to events from the Event Channel and dispatches an action to update the file upload progress in the Redux store.